C++ for Beginners & Professionals: In-Depth Online Course
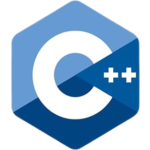
C++ Module
Course Title: Comprehensive C++ Programming
Module 1: Introduction to C++
- Overview of C++ programming language
- History and evolution of C++
- Setting up a development environment: IDEs, compilers
- Writing and running a simple C++ program
- Understanding the structure of a C++ program
- Basic input/output operations
Module 2: C++ Basics
- Basic syntax and data types in C++
- Variables, constants, and data manipulation
- Control flow: if statements, loops, switch statements
- Functions and parameter passing
- Understanding pointers and memory management
- Arrays and strings in C++
Module 3: Object-Oriented Programming (OOP) in C++
- Concepts of object-oriented programming
- Classes and objects in C++
- Data members and member functions
- Constructors and destructors
- Encapsulation and access specifiers
- Inheritance and polymorphism
Module 4: Advanced C++ Features
- Function overloading and operator overloading
- Static members and static functions
- Friend functions and friend classes
- Templates and generic programming
- Exception handling with try-catch blocks
- Smart pointers for memory management
Module 5: Standard Template Library (STL)
- Overview of the Standard Template Library (STL)
- Containers: vectors, lists, maps, sets, queues, stacks
- Algorithms: sorting, searching, modifying
- Iterators and iterator-based operations
- Using STL containers and algorithms in practical scenarios
- Customizing and extending STL components
Module 6: File Handling and Input/Output Operations
- File handling in C++: opening, reading, writing, and closing files
- Input/output stream classes: ifstream, ofstream, stringstream
- Working with text files and binary files
- Serializing and deserializing data with file streams
- Error handling and exception management in file operations
- Practical exercises and projects involving file handling
Module 7: Memory Management
- Dynamic memory allocation and deallocation with new and delete operators
- Understanding memory leaks and memory fragmentation
- RAII (Resource Acquisition Is Initialization) principle
- Smart pointers: unique_ptr, shared_ptr, weak_ptr
- Custom memory allocators and allocators in STL containers
- Best practices for efficient memory management in C++
Module 8: Multithreading and Concurrency
- Introduction to multithreading concepts
- Creating and managing threads in C++
- Synchronization mechanisms: mutexes, condition variables, semaphores
- Race conditions and deadlock prevention
- Implementing parallel algorithms with C++11 concurrency features
- Practical exercises and projects involving multithreading
Module 9: Advanced C++ Techniques
- Lambda expressions and closures
- Move semantics and rvalue references
- Variadic templates and perfect forwarding
- Type deduction and decltype
- Using C++ for system programming and low-level development
- Exploring advanced language features and patterns
Module 10: Debugging and Testing C++ Applications
- Debugging techniques with IDEs and debuggers
- Writing unit tests with Google Test or Catch2 frameworks
- Test-driven development (TDD) principles
- Performance profiling and optimization techniques
- Handling exceptions and logging in C++ applications
- Practical exercises and projects to reinforce debugging and testing skills
- 3 Weeks
- Weekdays : Mon to Fri ( 1hr/day )
- Weekend: 2hrs/day
- Flexible Time
- Free Session Videos
- Course Completion Certificate
- Lifetime Customer Support
- Helping to Get a Job
- Resume Preparation