Master Swift Programming: Build Powerful iOS Apps Online
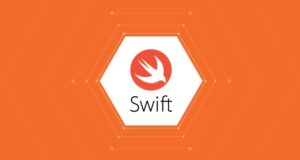
Swift Programming Module
Course Title: Comprehensive Swift Programming
Module 1: Introduction to Swift
- Overview of Swift programming language
- History and evolution of Swift
- Setting up a development environment: Xcode, Swift Playgrounds
- Writing and running a simple Swift program
- Understanding Swift syntax and basic data types
- Introduction to Swift playgrounds for interactive learning
Module 2: Swift Basics
- Variables, constants, and data manipulation in Swift
- Control flow: if statements, loops, switch statements
- Optionals and handling nil values
- Functions and parameter passing in Swift
- Working with arrays, dictionaries, and sets
- Introduction to tuples and pattern matching
Module 3: Object-Oriented Programming (OOP) in Swift
- Concepts of object-oriented programming
- Classes and structures in Swift
- Properties and methods in Swift classes
- Initialization and deinitialization
- Inheritance and overriding in Swift
- Value types vs. reference types in Swift
Module 4: Advanced Swift Features
- Enumerations and associated values
- Closures and closure expressions in Swift
- Higher-order functions and functional programming concepts
- Generics and generic programming in Swift
- Protocol-oriented programming (POP) in Swift
- Error handling with try-catch blocks and throwing functions
Module 5: Swift Standard Library
- Overview of the Swift Standard Library
- Collection types: arrays, dictionaries, sets
- String manipulation and formatting
- Working with dates and times
- File handling and input/output operations
- Practical exercises using Swift Standard Library components
Module 6: Asynchronous Programming in Swift
- Understanding asynchronous programming concepts
- Grand Central Dispatch (GCD) for concurrency
- Working with DispatchQueue for async tasks
- Handling asynchronous operations with closures
- Introduction to async/await in Swift
- Practical exercises and projects involving asynchronous programming
Module 7: Networking and Data Persistence
- Making HTTP requests with URLSession
- Parsing JSON data in Swift
- Encoding and decoding Codable objects
- Working with RESTful APIs in Swift
- Implementing data persistence with Core Data
- Storing and retrieving data from UserDefaults
Module 8: User Interface Development with SwiftUI
- Introduction to SwiftUI framework
- Creating user interfaces with SwiftUI’s declarative syntax
- Understanding views, modifiers, and layout in SwiftUI
- Handling user input with SwiftUI controls
- Navigation and presentation in SwiftUI apps
- Building dynamic and interactive UIs with SwiftUI
Module 9: iOS App Development with UIKit
- Overview of UIKit framework for iOS app development
- Creating iOS projects with Xcode and UIKit
- Working with view controllers, views, and controls
- Implementing navigation and tab bar interfaces
- Handling user interactions and gestures
- Introduction to Auto Layout for responsive UI design
Module 10: Advanced iOS Development
- Integrating Core Location for location-based services
- Using Core Animation for custom animations and transitions
- Implementing notifications and background tasks
- Working with multimedia: audio, video, and images
- Accessing device features: camera, accelerometer, gyroscope
- Deploying iOS apps to the App Store
Module 11: Debugging and Testing iOS Apps
- Debugging techniques with Xcode debugger
- Writing unit tests with XCTest framework
- Test-driven development (TDD) principles
- UI testing with XCTest UI framework
- Performance profiling and optimization techniques
- Handling exceptions and logging in iOS apps
- 1 to 1.5 Month
- Weekdays : Mon to Fri ( 1hr/day )
- Weekend: 2hrs/day
- Flexible Time
- Free Session Videos
- Course Completion Certificate
- Lifetime Customer Support
- Helping to Get a Job
- Resume Preparation